Maximum sum of hour glass in matrix
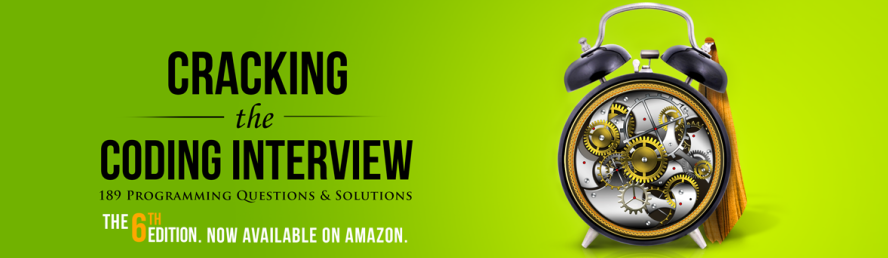
Problem:
Given a 6 x 6 2D array, arr
.
1 1 1 0 0 0 0 1 0 0 0 0 1 1 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0
An hourglass in A is a subset of values with indices facing in this pattern in arr
‘s graphical representation.
a b c d e f g
There are 16 hourglass in arr
. An hourglass is the sum of an hourglass values. Calculate the hourglass sum for every hourglass in arr
, then print maximum hourglass sum. The array always be 6x6
.
For example, for the below array,
-9 -9 -9 1 1 1 0 -9 0 4 3 2 -9 -9 -9 1 2 3 0 0 8 6 6 0 0 0 0 -2 0 0 0 0 1 2 4 0
The 16 hourglass sums are
-63, -34, -9, 12, -10, 0, 28, 23, -27, -11, -2, 10, 9, 17, 25, 18
The highest hourglass sum is 28 from the hourglass beginning at row 1, column 2.
0 4 3 1 8 6 6
Function Description:
Complete the function hourglassSum which has the following parameters.
int arr[6][6] : an array of intergers
Returns
int: the maximum hourglass sum
Input Format
Each of the 6 lines of inputs arr[i]
contains 6 space separated integers arr[i][j]
Constraints
-9 <= arr[i][j] <= 9 0 <=i, j<=5
Output Format:
Print the largest (maximum) hourglass sum found in arr
.
Sample Input
1 1 1 0 0 0 0 1 0 0 0 0 1 1 1 0 0 0 0 0 2 4 4 0 0 0 0 2 0 0 0 0 1 2 4 0
Output
19
Explanation:
All hour glasses are as below
1 1 1 1 1 0 1 0 0 0 0 0 1 0 0 0 1 1 1 1 1 0 1 0 0 0 0 0 0 1 0 1 0 0 0 0 0 0 0 0 1 1 0 0 0 0 2 0 2 4 2 4 4 4 4 0 1 1 1 1 1 0 1 0 0 0 0 0 0 2 4 4 0 0 0 0 0 2 0 2 0 2 0 0 0 0 2 0 2 4 2 4 4 4 4 0 0 0 2 0 0 0 1 0 1 2 1 2 4 2 4 0
The hour glass with maximum sum is below, and the sum is 19.
2 4 4 2 1 2 4
Solution
package org.span; import java.io.IOException; import java.util.Scanner; public class HourGlass { static int hourglassSum(int[][] arr) { int max = -99999, cur; for (int i = 0; i < 4; i++) { for (int j = 0; j < 4; j++) { cur = arr[i][j] + arr[i][j + 1] + arr[i][j + 2] + arr[i + 1][j + 1] + arr[i + 2][j] + arr[i + 2][j + 1] + arr[i + 2][j + 2]; if (cur > max) { max = cur; } } } return max; } private static final Scanner scanner = new Scanner(System.in); public static void main(String[] args) throws IOException { /*int[][] arr = { {1, 1, 1, 0, 0, 0}, {0, 1, 0, 0, 0, 0}, {1, 1, 1, 0, 0, 0}, {0, 0, 2, 4, 4, 0}, {0, 0, 0, 2, 0, 0}, {0, 0, 1, 2, 4, 0} };*/ int[][] arr = { {0, -4, -6, 0, -7, -6}, {-1, -2, -6, -8, -3, -1}, {-8, -4, -2, -8, -8, -6}, {-3, -1, -2, -5, -7, -4}, {-3, -5, -3, -6, -6, -6}, {-3, -6, 0, -8, -6, -7}, }; int result = hourglassSum(arr); System.out.println(result); } }